Just Do IT!
[React Native] react-navigation 본문
react-navigation란?
Routing and navigation for Expo and React Native apps. (출처: 공식문서)
react에서 router를 써서 다른 페이지로 이동했던 것처럼, 앱 내에서 다른 페이지로 넘어가게 만든다.
설치 방법
$ npm install @react-navigation/native
$ npx expo install react-native-screens react-native-safe-area-context
터미널에 두 명령어를 입력해서 설치해야 한다.
NavigationContainer
앱 상태를 관리하고 최상위 내비게이터를 앱 환경에 연결하는 역할
가장 최상위에서 모두 감싸서 이용한다. (import 해야 사용할 수 있음)
공식문서
https://reactnavigation.org/docs/navigation-container
https://reactnavigation.org/docs/navigation-container/
reactnavigation.org
Native Stack Navigator
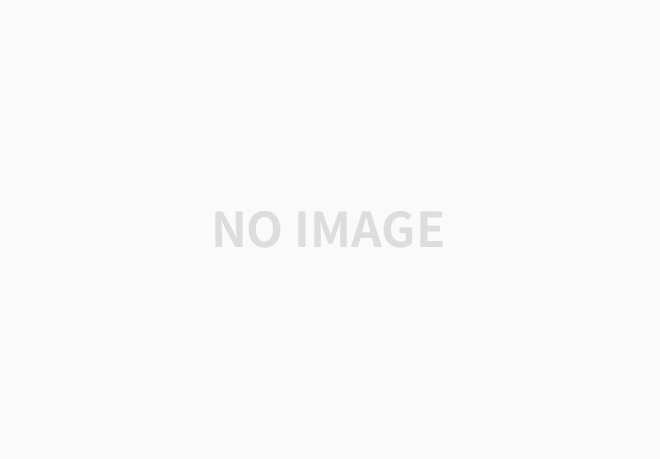
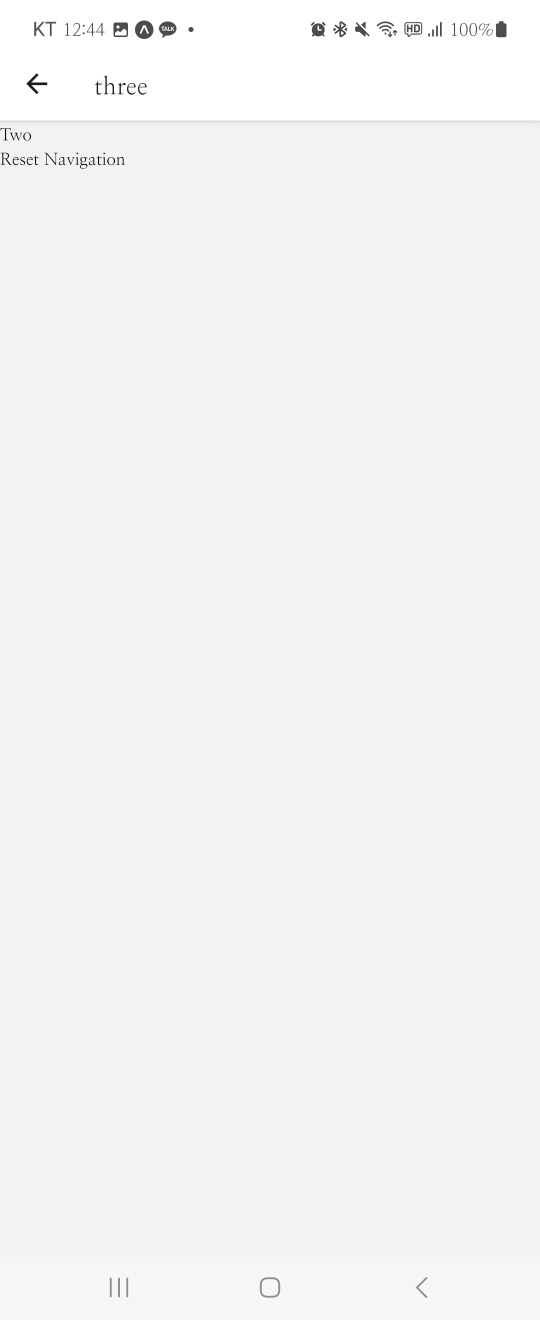
위의 사진처럼 Two를 클릭하면 다른 페이지로 이동할 수 있다.
공식문서 : https://reactnavigation.org/docs/native-stack-navigator/
https://reactnavigation.org/docs/native-stack-navigator/
reactnavigation.org
설치하기
$ npm install @react-navigation/native-stack
적용 예시
import { createNativeStackNavigator } from '@react-navigation/native-stack';
const Stack = createNativeStackNavigator();
export default function App() {
return (
<Stack.Navigator>
{/* initialRouteName : 맨 처음 나오는 화면을 설정할 수 있다 */}
{/* screenOptions : 객체를 인자로 받고 title 같은 것들을 바꿀 수 있다 */}
<Stack.Screen name="one" component={One} />
<Stack.Screen name="two" component={Two} />
<Stack.Screen name="three" component={Three} />
</Stack.Navigator>
);
}
- screen comonent는 기본적으로 navigation이라는 prop을 가지고 있다
- 여러 메소드 중 페이지 이동을 위해 navigate라는 메소드를 사용한다
- navigation prop 공식문서 통해 확인 가능 (reset, setOptions도 많이 쓴다)
- setOptions : 제목을 바꾼다거나 여러 가지 커스텀마이징 가능
- goBack : 뒤로가기
- reset: navigation history를 초기화시킨다 (뒤로가기 불가능)
const Three = ({ navigation: { goBack, reset } }) => {
return (
<>
<TouchableOpacity onPress={() => goBack()}>
<Text>Two</Text>
</TouchableOpacity>
<TouchableOpacity
onPress={() =>
reset({
index: 1,
routes: [{ name: "three" }, { name: "two" }],
})
}
>
<Text>Reset Navigation</Text>
</TouchableOpacity>
</>
);
};
Bottom Tabs Navigator
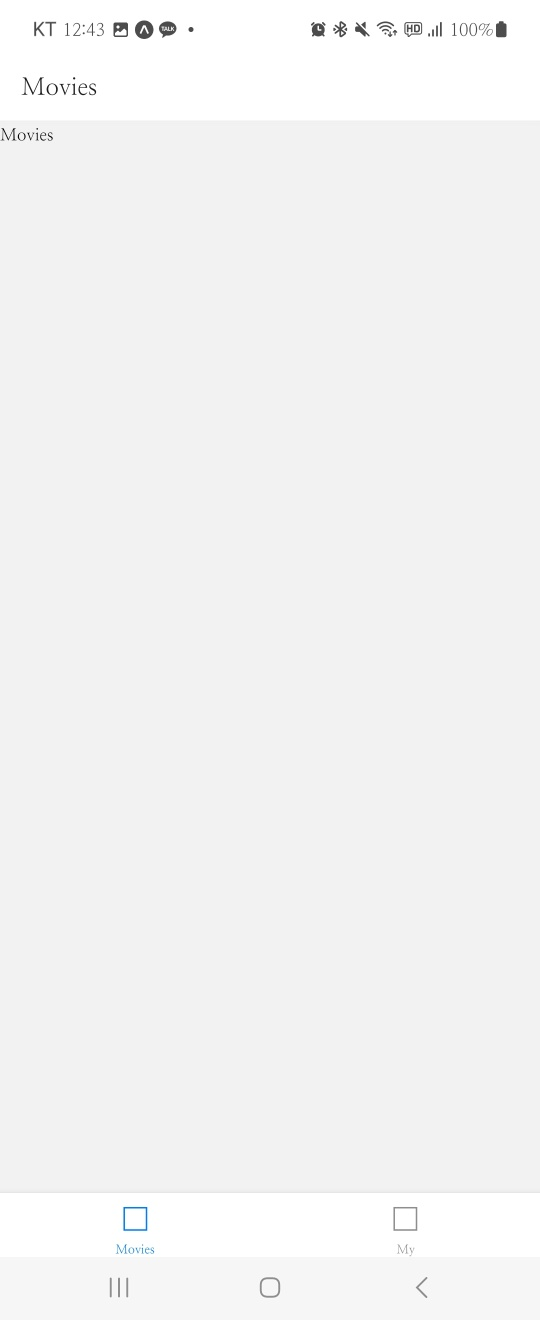
위의 예시처럼 앱의 하단에 고정되어있는 navigator (클릭 시 다른 페이지로 이동)
공식문서 : https://reactnavigation.org/docs/bottom-tab-navigator/
https://reactnavigation.org/docs/bottom-tab-navigator/
reactnavigation.org
설치하기
$ npm install @react-navigation/bottom-tabs
예시 코드
import React from "react";
import { createBottomTabNavigator } from "@react-navigation/bottom-tabs";
import Movies from "../screen/Movie";
import My from "../screen/My";
import { MaterialIcons } from "@expo/vector-icons";
import { Foundation } from "@expo/vector-icons";
const Tab = createBottomTabNavigator();
export default function Tabs() {return (
<Tab.Navigator
screenOptions={{
tabBarLabelPosition: "beside-icon",
}}
sceneContainerStyle={{ backgroundColor: DARK_COLOR }}
>
{/* initialRouteName : 맨 처음 나오는 화면을 설정할 수 있다 */}
{/* screenOptions : 객체를 인자로 받아서 제목 등을 변경할 수 있다 */}
{/* (각각에 options라는 prop을 주고 변경 가능) */}
{/* sceneContainerStyle : screen 화면에 대한 스타일링을 할 수 있는 속성 */}
<Tab.Screen
options={{
title: "영화",
headerTitleAlign: "center",
tabBarLabel: "Movies",
tabBarIcon: ({ color, size }) => (
<MaterialIcons name="movie" size={size} color={color} />
),
}}
name="Movies"
component={Movies}
/>
<Tab.Screen
options={{
title: "내가 작성한 댓글들",
headerTitleAlign: "center",
tabBarLabel: "My",
tabBarIcon: ({ color, size }) => (
<Foundation name="social-myspace" size={size} color={color} />
),
}}
name="My"
component={My}
/>
</Tab.Navigator>
);
}
Stacks 와 Tabs 같이 사용하기
navigation 폴더에 새로운 Root.js 파일 생성
import React from "react";
import { createNativeStackNavigator } from "@react-navigation/native-stack";
import Tabs from "./Tabs";
import Stacks from "./Stacks";
const Stack = createNativeStackNavigator();
export default function Root() {
return (
<Stack.Navigator screenOptions={{ headerShown: false }}>
<Stack.Screen name="Tabs" component={Tabs} />
<Stack.Screen name="Stacks" component={Stacks} />
</Stack.Navigator>
);
}
App.js에 Root 추가
import Root from "./navigation/Root";
export default function App() {
return (
<NavigationContainer>
<Root />
</NavigationContainer>
);
}
이런 형식으로 추가하면 Stack과 Tab을 같이 사용할 수 있다.
'개발 공부 > React Native' 카테고리의 다른 글
[React Native] ScrollView 대신 FlatList 사용하기 (0) | 2023.01.04 |
---|---|
[React Native] 영화 정보 API 사용하기 (TMDB) (0) | 2023.01.04 |
[React Native] react native 프로젝트와 firebase 연동하기 (1) | 2023.01.02 |
[React Native] AsyncStorage 사용법 (0) | 2022.12.30 |
[React Native] Expo 사용법 (0) | 2022.12.29 |